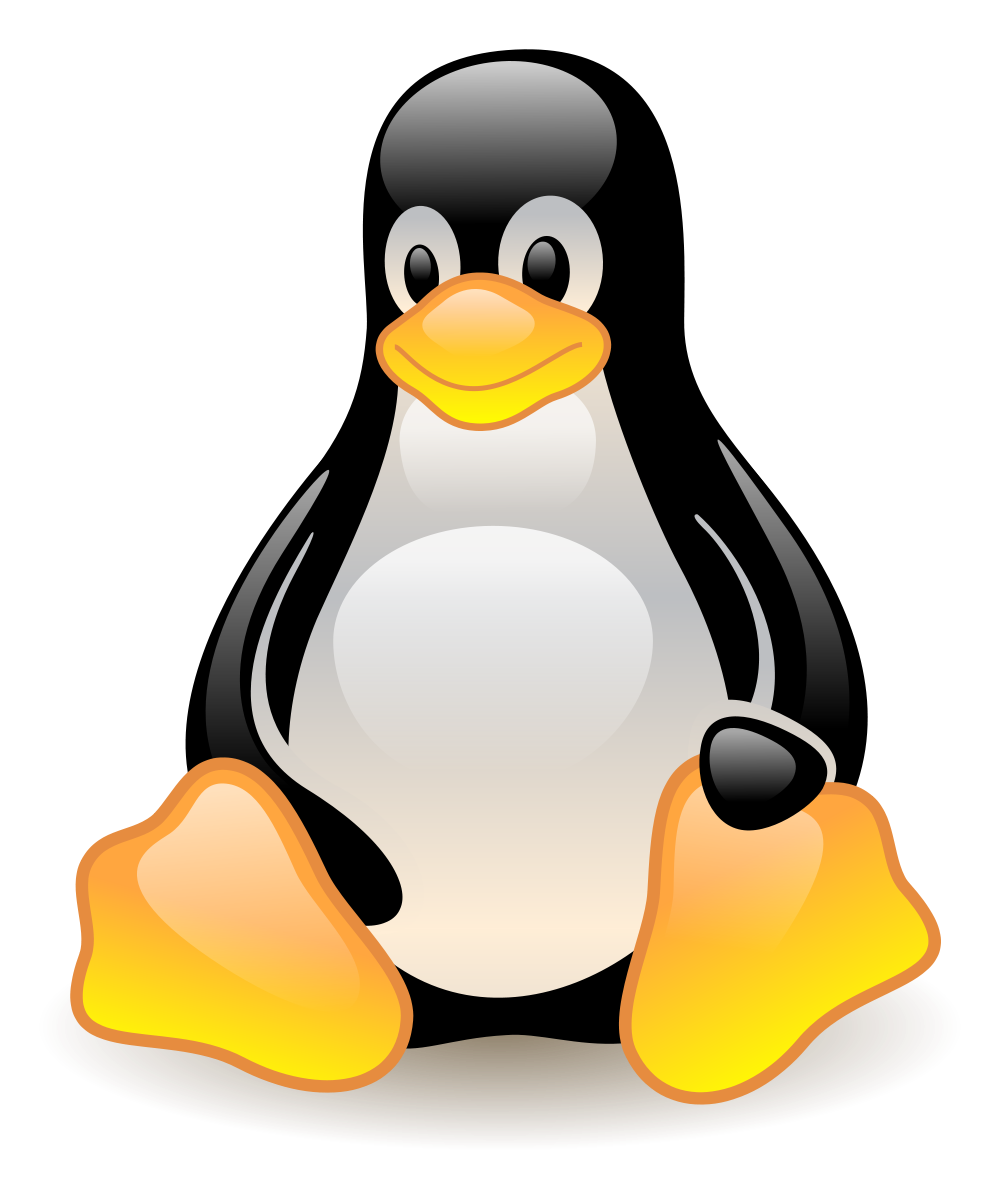
Voltage
July 1, 2023, 9:12 a.m.
Error handling in Python: The Correct way to handle errors in python.
Introduction.
Few days back, a fellow python enthuasist came to me inquiring on the best way to implement error handling in python.
Programmer: "Well, do you belive in yourself?"
Me: "Go ahead and spit your need out, it will choke you!"
Programmer: "Show me techniques on implementing error handling!"
What a silly question in the morning!
Exceptions and Error Handling in Python.
Just like any other programming language, python has mechanisms to handle failing safely when a program executions fails. Imagine you have a programme to take an input from the user below:
number = int(input("[+] Enter year: "))
From the code above, we are simply taking a user input in form of an integer. The next question is, what if a user enters something not an integer? well, we will end up with bunch of errors as below.
Output.
number = int(input("[+] Enter year: "))
[+] Enter year: rat
Traceback (most recent call last):
File "<pyshell#2>", line 1, in <module>
number = int(input("[+] Enter year: "))
ValueError: invalid literal for int() with base 10: 'rat'
Well, this is so shouty, the code is complaining that it doesn't understand whatever it is that the user keyed in. If you are a programmer, you will easily understand and debug the code, however, if you have no technical expertise in the field of programming, you will fail to understand what should fix this.
Now, how can we implement an exception handling such that the program fails well without throwing these errors?
1. Use a general Error handling technique to capture any errors encountered during input.
The code below shows a general way of capturing any errors encountered.
try:
number = int(input("[+] Enter year: "))
print("[+] Year captured successfully!")
except Exception as e:
print("[-] ERROR: " + str(e))
Output
[+] Enter year: 2010
[+] Year captured successfully!
Hoping that you don't enter something "stupid", you get an output above, however, if you try to test waters, you get an output below.
[+] Enter year: abc
[-] ERROR: invalid literal for int() with base 10: 'abc'
From the output above, we have properly captured any error that could be associated with the input from the user.
2. Capturing specific error.
The next technique of capturing the error is by directly pointing of the possible error likely to be encountered and printing it. In our first attempt to capture user input, we got ValueError. Now we can capture this error specifically without having to try and capture any possible error that could aries.
try:
number = int(input("[+] Enter year: "))
print("[+] Year captured successfully!")
except ValueError:
print("[-] You entered none integer")
Output.
From this code, we are very specific that we only want to capture an error if it is a ValueError. This mean it is not an integer as was expected. We then print the correct error message.
[+] Enter year: abc
[-] You entered none integer
Best Practices.
Depending on your scenario, either of this techniques could work however, I would recommend capturing general errors i.e the first option. This will save you alot of pain looking for a specific error in in a thousands line of code program. Just capture any possible error that could be associated with whatever it is that you are trying to validate.
Conclusion.
That's all for today forks, the sun will rise and we will again tomorrow computer geeks.