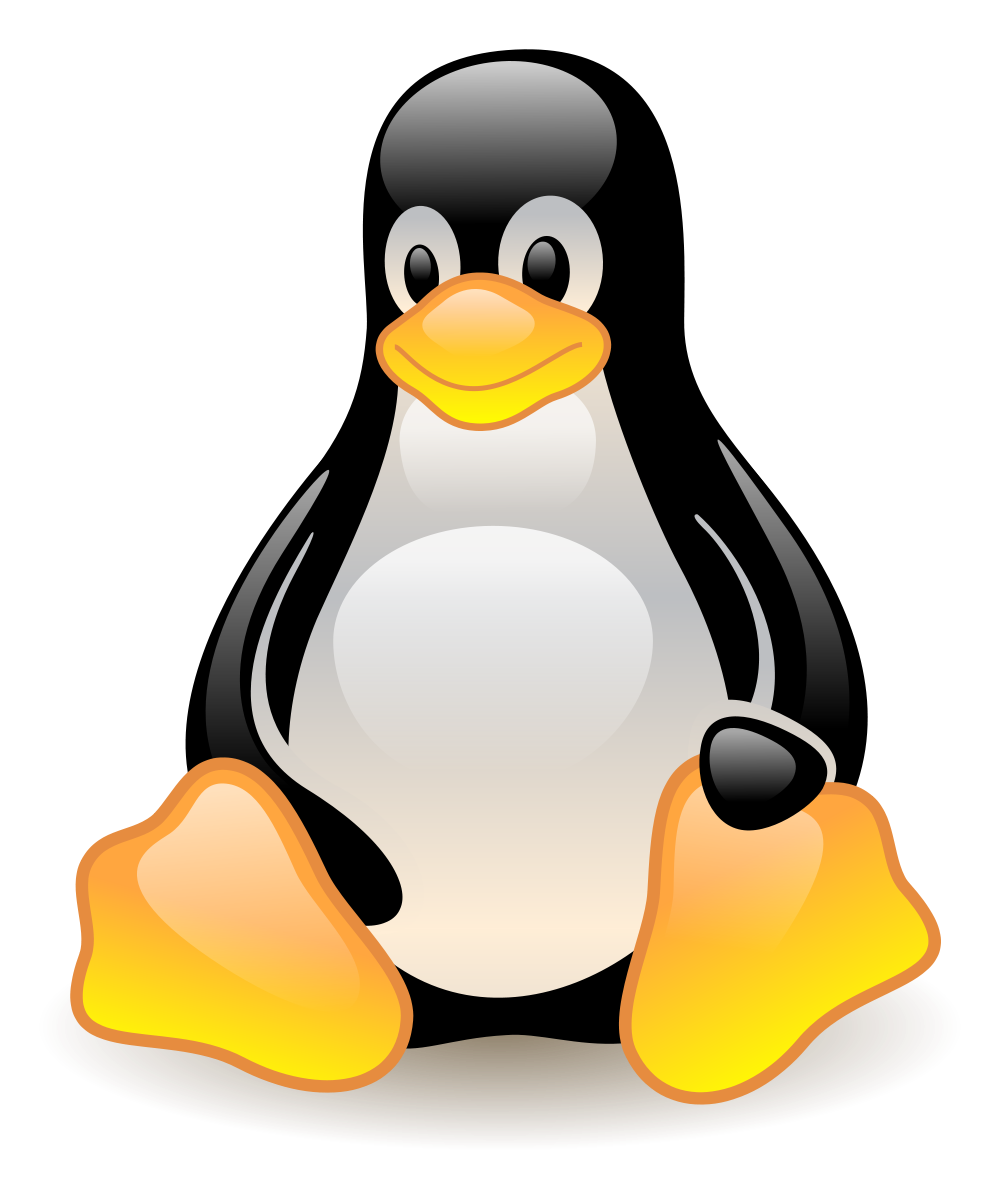
Voltage
Oct. 2, 2023, 2:15 p.m.
Improving productivity and Saving development time in Django.
Introduction.
Welcome back my fellow tech enthuasists and prgrammers. It has been a while since I last communicated however, I am here today to let you know of appropriate ways on which you can improve your productivity as a Django developer and increase your development speed and delivery of cutting edge software. Often, developers are under tight deadlines to deliver projects. Django is one of the best frameworks that improves developer's flexibility and lowers the development time taken to complete a software, however given that Django already saves on developer's time, we can lower the time taken to develop a software and release it further. The following are some of the best ways on how you can lower your development time.
Use Context Processors.
Context processors are the best way to make variables and database information available across all web pages in an application. Assuming that your developed webpage already have series of webpages and you need to show a user profile image across several templates/web pages, without having to write the profile context on every view for every template, you can write a single profile context on a single file called context processor for instance context_processor.py. The you can go ahead and add your contxet processor under the context processors section in your settings.py. The following code shows how to write a simple contxet processor to display profile data from profile model assuming that you have such a model in your database.
def user_profile_context_processor(request):
""" Adds the user's profile data to the template context."""
if request.user.is_authenticated:
profile = User.objects.get(pk=request.user.pk).profile
else:
profile = None return { 'profile': profile, }
You should then add the context processor on your settings.py file as below.
# settings.py
TEMPLATE_CONTEXT_PROCESSORS = [
... 'my_project.context_processors.user_profile_context_processor',
]
On one of your templates, you may access the profile data attributes as below.
{% if profile %}
<h1>{{ profile.first_name }} {{ profile.last_name }}</h1>
<p>{{ profile.bio }}</p>
{% else %}
<p>You are not logged in.</p> {% endif %}
Use Base Template.
In most situations in your development carrier, you will have to use the same navigation bar across all your webpages. In certain situations, you will have two options. The first option will be to write a single html ltemplate that contains all your navbar code and then extend this template across all other templates. The second option will be to copy and paste the navbar content across each and every template in your application. This approach will not only require more time but will also break one of the important Python rules, DO Not Repeat Yourself(DRY). This will create a code that is hard to maintain and scale. Assuming at some point you need to print a user name on the navbar alongside a welcome message, you will walk through every template adding this logic. The chances are, you will end up forgetting even certain templates if your application has a bigger codebase. Learn to use reusable template tags. The following code shows a simple implementation on how you can create a single base.html template and extend it across all other templates.
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
</head>
<body>
<header>
<h1>My Website</h1>
<nav>
<a href="/">Home</a>
<a href="/about/">About</a>
<a href="/contact/">Contact</a>
</nav>
</header>
<main>
{% block content %}
<!-- This is where the content of each page will go. -->
{% end blockcontent %}
</main>
<footer>
<p>Copyright © 2023 My Website</p>
</footer>
</body>
</html>
In one of your templates, you may extend the base template as below.
{% extends 'base.html' %}
{% block content %}
<h1>About My Website</h1>
<p>This is a simple website that I created using Django.</p>
{% endblock %}
Use Frontend Styling Library.
To help speed up your development, use a dedicated front end styling css library, you may use bootstrap, tailwind css, material ui amongst others depending on your preference. This will lower the amount of time you will take writing css code that will take care of both smaller screens and bigger screens. By using a dedicated css framework, your work will be easier when it comes writing response web applications. This will improve your productivity and lower your developoment time.
Conclusions.
Having gone through ways of lowering the amount of time you will take writing code, dive into class and learn the best practices associated with the techniques shared above. For instance take much time learning about context processors to avoid writing broken code and insecure code. Context processors can easilky expose your database data to unauthorized persons if inappropriately used. Thank you until next time.
Voltage, ([email protected])